The Java collections framework is a set of interfaces and classes that provide various data structures and algorithms for storing and manipulating data. These collections include lists, sets, maps, and queues, among others. Understanding the collections framework is an important aspect of Java programming and is often tested in interviews. In this article, I will cover some common and advanced Java collections interview questions that you may encounter in a technical interview.
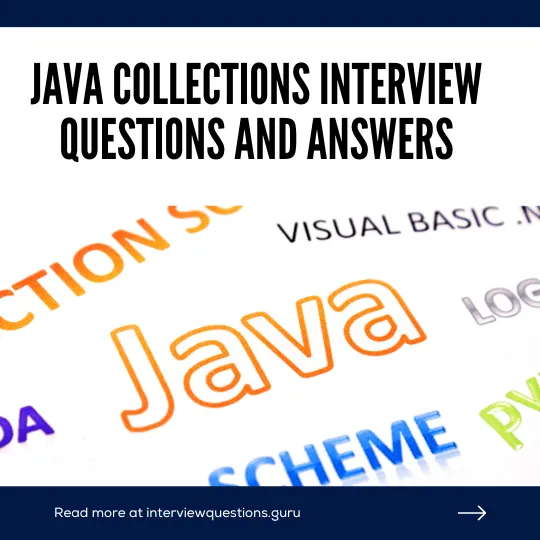
Table of Contents
Common Java collections Interview Questions and Answers
What is the difference between an array and an ArrayList in Java?
An array is a fixed-size data structure that holds a sequence of elements of the same type. An ArrayList, on the other hand, is a resizable array implementation of the List interface. It has the ability to grow and shrink dynamically to accommodate the number of elements. ArrayLists also provide additional methods for inserting, deleting, and manipulating elements, such as add(), remove(), and get().
How does a HashMap work in Java?
A HashMap is a implementation of the Map interface that stores key-value pairs in a hash table. When you insert a key-value pair into a HashMap, the key is hashed and the resulting hash code is used to determine the index in the hash table where the value will be stored. To retrieve a value, the HashMap calculates the hash code of the key and uses it to look up the value in the hash table.
How do you implement a queue using a linked list in Java?
A queue is a First-In-First-Out (FIFO) data structure, and a linked list is a linear data structure that consists of a chain of nodes. To implement a queue using a linked list, you can create a class that has two fields: a “head” field to represent the front of the queue and a “tail” field to represent the back of the queue. To add an element to the queue (enqueue), you can create a new node with the element and add it to the back of the linked list (at the tail). To remove an element from the queue (dequeue), you can remove the front node of the linked list (at the head).
What is the difference between a Set and a List in Java?
A Set is a collection that does not allow duplicate elements and does not have a specific order. A List, on the other hand, is an ordered collection that can contain duplicate elements. Sets are commonly used when you want to store a unique set of elements, while Lists are useful when you need to preserve the order of the elements and/or allow duplicates.
Complete Basic Java Interview Questions and Answers with Tips
What are the collection classes in Java?
The Java collections framework consists of several interfaces and classes that provide various data structures and algorithms for storing and manipulating data. Here is a list of the main interfaces and classes in the Java collections framework:
Interfaces:
- Collection: a root interface that represents a group of objects known as its elements.
- List: an ordered collection that allows duplicates.
- Set: a collection that does not allow duplicates.
- Map: a collection that maps keys to values.
- Queue: a collection that orders elements in a FIFO (First-In-First-Out) manner.
Classes:
- ArrayList: a resizable array implementation of the List interface.
- LinkedList: a doubly-linked list implementation of the List and Queue interfaces.
- HashSet: a hash table implementation of the Set interface.
- TreeSet: a sorted set implementation based on a tree data structure.
- HashMap: a hash table implementation of the Map interface.
- TreeMap: a sorted map implementation based on a tree data structure.
- PriorityQueue: a priority queue implementation based on a heap data structure.
These are just a few examples of the classes and interfaces available in the Java collections framework. There are many more, each with its own characteristics and use cases.
Which collection is the fastest in Java?
The performance of a collection depends on several factors, including the size of the collection, the operations being performed, and the hardware and JVM (Java Virtual Machine) being used. Here is a rough comparison of the time complexity of some common operations for some of the main Java collections:
ArrayList:
- Insertion at end: O(1)
- Insertion at beginning or middle: O(n)
- Access by index: O(1)
- Search: O(n)
- Deletion by index: O(n)
LinkedList:
- Insertion at end or beginning: O(1)
- Insertion at middle: O(1) (assuming reference to the node is available)
- Access by index: O(n)
- Search: O(n)
- Deletion by index: O(n)
HashSet:
- Insertion: O(1) (on average)
- Access: O(1) (on average)
- Search: O(1) (on average)
- Deletion: O(1) (on average)
TreeSet:
- Insertion: O(log n)
- Access: O(log n)
- Search: O(log n)
- Deletion: O(log n)
HashMap:
- Insertion: O(1) (on average)
- Access: O(1) (on average)
- Search: O(1) (on average)
- Deletion: O(1) (on average)
TreeMap:
- Insertion: O(log n)
- Access: O(log n)
- Search: O(log n)
- Deletion: O(log n)
As you can see, the time complexity of each operation varies depending on the collection. In general, HashSet and HashMap have better performance for insertions, access, search, and deletion compared to the other collections, due to their use of a hash table data structure. However, they do not preserve the order of the elements. If you need to preserve the order, you can use a LinkedList or a TreeSet/TreeMap, which have slower performance but offer order-based operations.
When do you use Set, List, and Map in Java?
In Java, the Set, List, and Map interfaces are part of the collections framework and are used to store and manipulate groups of objects. Here are some general guidelines for when to use each interface:
- Set: A Set is a collection that does not allow duplicate elements and does not have a specific order. It is useful when you want to store a unique set of elements and do not care about the order. Examples of set implementations include HashSet and TreeSet.
- List: A List is an ordered collection that allows duplicates. It is useful when you need to preserve the order of the elements and/or allow duplicates. Examples of list implementations include ArrayList and LinkedList.
- Map: A Map is a collection that maps keys to values. It is useful when you want to store key-value pairs and retrieve values based on their keys. Examples of map implementations include HashMap and TreeMap.
In summary, use a Set when you want to store a unique set of elements, use a List when you need to preserve the order of the elements and/or allow duplicates, and use a Map when you want to store key-value pairs and retrieve values based on their keys.
What is the difference between Comparator and Comparable in Java?
In Java, the Comparable interface is used to define the natural ordering of a class, while the Comparator interface is used to define an alternative ordering of a class.
Here are some key differences between Comparable and Comparator:
- Implementation: Comparable is implemented by a class that wants to be sorted, while Comparator is implemented by a separate class that is used to compare objects.
- Method: Comparable defines a single method called compareTo(), while Comparator defines two methods: compare() and equals().
- Natural ordering: Comparable defines the natural ordering of a class, which means that the ordering is inherent to the class and cannot be changed. Comparator, on the other hand, provides an alternative ordering that can be changed.
- Use: Comparable is used to sort a list of objects of a single class, while Comparator is used to sort a list of objects of different classes or to provide multiple sorting options for a single class.
What is the “diamond” operator in Java?
In Java, the “diamond” operator is used in conjunction with generic types to improve code readability. It allows you to infer the type of a generic class or interface based on the context in which it is used.
Here’s an example of how the diamond operator can be used:
Map<String, List<String>> map = new HashMap<>();
In this example, the type of the map variable is Map<String, List<String>>, and the type of the object being instantiated is HashMap<>. The type of the object being instantiated is inferred from the type of the variable, so you don’t have to specify it again.
Without the diamond operator, the code would look like this:
Map<String, List<String>> map = new HashMap<String, List<String>>();
As you can see, the diamond operator helps to make the code more concise and easier to read. It was introduced in Java 7.
What is the covariant method overriding in Java?
In Java, covariant method overriding is a way to override a method in a subclass in a way that allows the return type of the subclass method to be a subclass of the return type of the superclass method. This is possible because of the way that Java handles generics and type parameters.
Here’s an example of covariant method overriding in Java:
class Animal {
public Animal getAnimal() {
return this;
}
}
class Cat extends Animal {
@Override
public Cat getAnimal() {
return this;
}
}
In this example, the Cat class overrides the getAnimal()
method of the Animal class, and the return type of the Cat class’s getAnimal()
method is Cat, which is a subclass of Animal. This is an example of covariant method overriding, because the return type of the subclass method is a subclass of the return type of the superclass method.
Covariant method overriding can be a useful feature in certain situations, but it is important to use it carefully, as it can also introduce potential type errors into your code if not used correctly.
What is DeQueue?
A deque, also known as a double-ended queue, is a data structure that allows you to add and remove elements from both the front and the back of the queue. It is similar to a queue, which only allows you to add elements to the back and remove them from the front, but a deque allows you to do both of these operations at both ends of the queue.
Deques are often implemented as a circular buffer, which allows for efficient insertion and removal of elements at both ends of the queue. They are commonly used as a data structure for implementing stacks and queues, and they can also be used for other purposes such as implementing a traversal of a binary tree.
In Java, the Deque interface is part of the Java Collection framework and extends the Queue interface. It defines methods for adding, removing, and examining elements at both ends of the deque. The ArrayDeque class is a widely used implementation of the Deque interface that is backed by a resizable array.
Is LinkedList in Java a doubly or singly linked list?
In Java, LinkedList is a doubly linked list data structure.
Advanced Java collections interview questions and answers
How do you implement a priority queue using a heap in Java?
A priority queue is a queue data structure that allows each element to have a priority associated with it, and the element with the highest priority is always removed first. A heap is a complete binary tree that satisfies the heap property, which states that the value of each node is greater than or equal to the values of its children (for a max-heap) or less than or equal to the values of its children (for a min-heap).
To implement a priority queue using a heap in Java, you can create a class that has an array field to store the heap elements and an integer field to represent the size of the heap. To add an element to the priority queue (offer), you can check if the heap is full (size==capacity) and, if not, add the element to the end of the array and then percolate it up to its correct position according to its priority.
To remove the highest priority element from the priority queue (poll), you can check if the heap is empty (size==0) and, if not, remove the root element from the array, replace it with the last element of the array, and then percolate it down to its correct position according to its priority.
What is the difference between fail-fast and fail-safe iterators?
Fail-fast iterators throw a ConcurrentModificationException
if they detect that the underlying collection has been modified while they are iterating over it. Fail-safe iterators, on the other hand, do not throw an exception and continue to work even if the underlying collection has been modified. Fail-fast iterators are faster and more efficient, but they are not thread-safe. Fail-safe iterators are thread-safe, but they may not reflect the latest state of the collection if it is being modified concurrently.
What is the difference between a TreeSet and a TreeMap?
A TreeSet is a sorted set data structure that is implemented using a tree. It stores a set of elements and allows you to perform operations such as adding, removing, and searching for elements in the set. A TreeMap is a map data structure that is also implemented using a tree. It stores key-value pairs and allows you to perform operations such as inserting, deleting, and searching for key-value pairs in the map. The main difference between the two is that a TreeSet only stores elements, while a TreeMap stores key-value pairs.
What is the difference between a HashSet and a LinkedHashSet?
Both HashSet and LinkedHashSet are implementations of the Set interface and use a hash table to store their elements. The main difference between the two is that a HashSet does not maintain the order of its elements, while a LinkedHashSet maintains the insertion order of its elements. This means that the elements in a LinkedHashSet are iterated in the order in which they were added to the set, while the elements in a HashSet are not iterated in any particular order.
What is the difference between a ArrayList and a LinkedList?
Both ArrayList and LinkedList are implementations of the List interface and are used to store and manipulate lists of objects. The main difference between the two is how they store their elements. An ArrayList uses an array to store its elements, while a LinkedList uses a linked list data structure. As a result, ArrayList is faster at accessing and retrieving elements, but slower at inserting and deleting elements, compared to LinkedList. LinkedList, on the other hand, is faster at inserting and deleting elements, but slower at accessing and retrieving elements compared to ArrayList.
Can HashMap have duplicate keys?
No, a HashMap cannot have duplicate keys. The HashMap class is a map implementation that uses a hash table to store its key-value pairs. Each key in a HashMap is unique, and trying to add a new key-value pair with a key that is already present in the map will overwrite the value associated with that key.
Here’s an example of how this works:
Map<String, Integer> map = new HashMap<>(); map.put("apple", 1); map.put("banana", 2); map.put("apple", 3); System.out.println(map.get("apple")); // prints 3
In this example, we add three key-value pairs to the map, with the keys “apple”, “banana”, and “apple”. The first two key-value pairs are added successfully, but when we try to add the third key-value pair with the key “apple”, it overwrites the value associated with the key “apple” in the map, so the value of the “apple” key becomes 3.
Is HashMap linear or nonlinear?
A HashMap
is a nonlinear data structure. It is implemented using a hash table, which is a data structure that uses a hash function to map keys to indices in an array. The hash function maps keys to indices in a way that is designed to distribute the keys evenly across the array, which allows for efficient insertion, deletion, and retrieval of key-value pairs in the map.
However, because the hash function is not a perfect function, there may be collisions, where two or more keys are mapped to the same index in the array. To handle collisions, HashMap
uses a technique called chaining, where the elements that map to the same index are stored in a linked list at that index.
Because a HashMap
uses a nonlinear data structure and may involve collisions and chaining, it does not have a linear order, unlike data structures such as arrays and linked lists, which do have a linear order.
What is the difference between a linked hash map and a PriorityQueue
LinkedHashMap and PriorityQueue are two very different data structures in Java.
LinkedHashMap is a map data structure that is implemented using a hash table and a linked list. It stores key-value pairs and allows you to perform operations such as inserting, deleting, and searching for key-value pairs in the map. One of the main features of LinkedHashMap is that it maintains the insertion order of its elements, meaning that the elements are iterated in the order in which they were added to the map.
PriorityQueue, on the other hand, is a queue data structure that is implemented using a heap. It stores elements and allows you to add and remove elements from the queue. One of the main features of PriorityQueue is that it is a priority queue, meaning that the elements in the queue are ordered according to their natural ordering or according to a Comparator provided at the time of creation. The element at the front of the queue is always the one with the highest priority.
So, the main differences between LinkedHashMap and PriorityQueue are:
LinkedHashMap is a map data structure, while PriorityQueue is a queue data structure.
LinkedHashMap maintains the insertion order of its elements, while PriorityQueue orders its elements according to their priority.
LinkedHashMap is implemented using a hash table and a linked list, while PriorityQueue is implemented using a heap.